12 - Sprite Props
To avoid our game auto-starting every time it loads, we need a way to pause the game, for example when in a menu screen. We can do this by passing props
between Sprites.
In our Level
Sprite we can setup a paused
prop which we access in the loop
method. Pausing is simple: we just return early with the current state
to avoid any of the game logic running.
Then in our top-level Game
Sprite we add some state to know if we're viewing the menu or not. If we are, we pause the level by passing the paused
prop in.
- JavaScript
- TypeScript
1import { makeSprite, t } from "@replay/core";
2import { Bird } from "./bird";
3
4const birdX = 0;
5
6export const Level = makeSprite({
7 init() {
8 return {
9 birdY: 10,
10 birdGravity: -12,
11 };
12 },
13
14 loop({ props, state, getInputs }) {
15 if (props.paused) {
16 return state;
17 }
18
19 const inputs = getInputs();
20
21 let { birdGravity, birdY } = state;
22
23 birdGravity += 0.8;
24 birdY -= birdGravity;
25
26 if (inputs.pointer.justPressed || inputs.keysJustPressed[" "]) {
27 birdGravity = -12;
28 }
29
30 return {
31 birdGravity,
32 birdY,
33 };
34 },
35
36 render({ state, device }) {
37 const { size } = device;
38 return [
39 t.rectangle({
40 color: "#add8e6",
41 width: size.width + size.widthMargin * 2,
42 height: size.height + size.heightMargin * 2,
43 }),
44 Bird({
45 id: "bird",
46 x: birdX,
47 y: state.birdY,
48 rotation: Math.max(-30, state.birdGravity * 3 - 30),
49 }),
50 ];
51 },
52});
53
1import { makeSprite, t } from "@replay/core";
2import { WebInputs } from "@replay/web";
3import { iOSInputs } from "@replay/swift";
4import { Bird } from "./bird";
5
6const birdX = 0;
7
8type LevelProps = {
9 paused: boolean;
10};
11
12type LevelState = {
13 birdY: number;
14 birdGravity: number;
15};
16
17export const Level = makeSprite<LevelProps, LevelState, WebInputs | iOSInputs>({
18 init() {
19 return {
20 birdY: 10,
21 birdGravity: -12,
22 };
23 },
24
25 loop({ props, state, getInputs }) {
26 if (props.paused) {
27 return state;
28 }
29
30 const inputs = getInputs();
31
32 let { birdGravity, birdY } = state;
33
34 birdGravity += 0.8;
35 birdY -= birdGravity;
36
37 if (inputs.pointer.justPressed || inputs.keysJustPressed[" "]) {
38 birdGravity = -12;
39 }
40
41 return {
42 birdGravity,
43 birdY,
44 };
45 },
46
47 render({ state, device }) {
48 const { size } = device;
49 return [
50 t.rectangle({
51 color: "#add8e6",
52 width: size.width + size.widthMargin * 2,
53 height: size.height + size.heightMargin * 2,
54 }),
55 Bird({
56 id: "bird",
57 x: birdX,
58 y: state.birdY,
59 rotation: Math.max(-30, state.birdGravity * 3 - 30),
60 }),
61 ];
62 },
63});
64
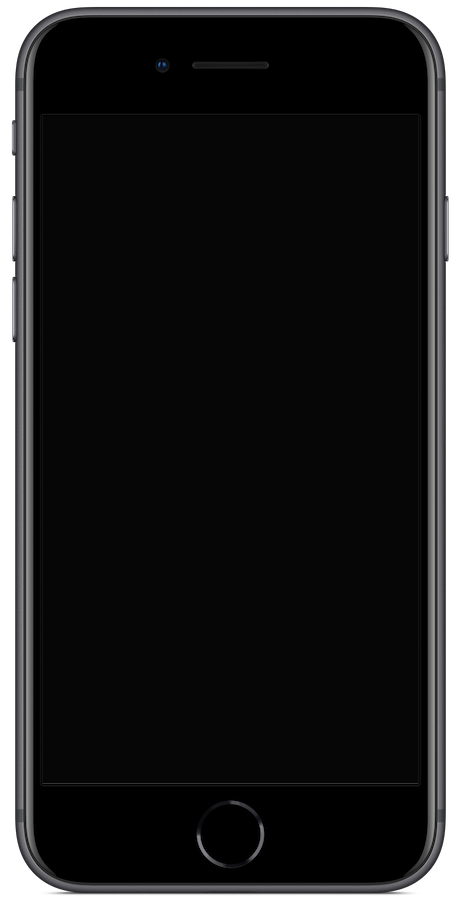