4 - Game Props
Below the Game
are the gameProps
. We don't apply them now, but when we run the game on web or iOS, we'll import the Game
and its gameProps
and apply them like Game(gameProps)
.
You can see an example of this in the web/index
file, where we call the renderCanvas
function from the @replay/web
package to render our game in the browser.
gameProps
requires an id
for the Game
Sprite and the size
of our game, which we'll discuss next. We also add an optional defaultFont
prop
to set the default font size and keep a consistent font family for any text we render later on.
- JavaScript
- TypeScript
1import { makeSprite } from "@replay/core";
2import { Bird } from "./bird";
3
4export const Game = makeSprite({
5 render() {
6 return [
7 Bird({
8 id: "bird",
9 }),
10 ];
11 },
12});
13
14export const gameProps = {
15 id: "Game",
16 size: {
17 width: 400,
18 height: 600,
19 },
20 defaultFont: {
21 family: "Helvetica",
22 size: 24,
23 },
24};
25
1import { makeSprite, GameProps } from "@replay/core";
2import { Bird } from "./bird";
3
4export const Game = makeSprite<GameProps>({
5 render() {
6 return [
7 Bird({
8 id: "bird",
9 }),
10 ];
11 },
12});
13
14export const gameProps: GameProps = {
15 id: "Game",
16 size: {
17 width: 400,
18 height: 600,
19 },
20 defaultFont: {
21 family: "Helvetica",
22 size: 24,
23 },
24};
25
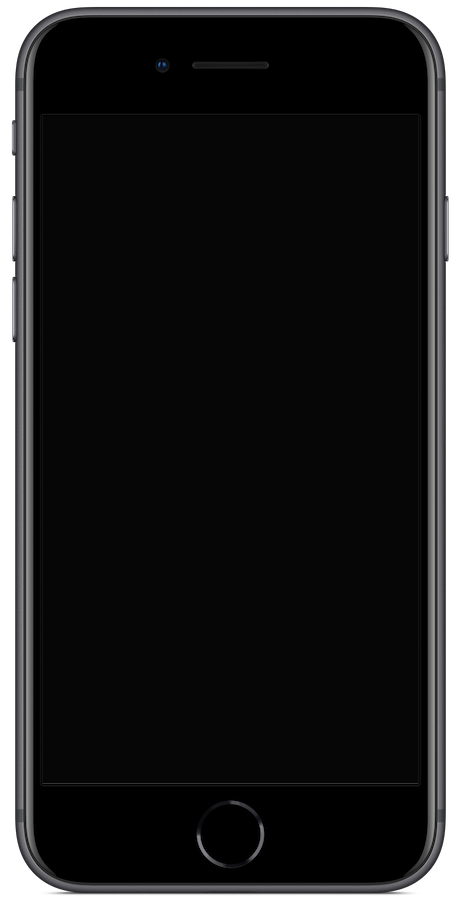