20 - Setup Tests
The @replay/test
package provides a platform to automate gameplay tests for our game. We can write our tests as though we're playing the game for real, without needing to know much about the internal workings of our code.
Using Jest we can write an initial test to confirm we can start the game.
In __tests__/game.test
replace what's there with the code on the right. We pass our Game
Sprite (and gameProps
) into the testSprite
function, which returns some more useful functions for inspecting our game. Since the test platform doesn't know if we want to run on web or iOS, we need to supply the inputs we'd expect on those platforms through initInputs
.
Then we have to wait for our loading screen to pass by calling await resolvePromises()
.
Below that getByText(mainMenuText)
searches all t.text
Textures that match the string passed in. This confirms that our main menu is visible on the initial render.
Next we call updateInputs
to simulate a mouse click or tap to start, then progress one frame with nextFrame
. After that we reset the inputs and progress one more frame. Now that the game's started, we confirm in our test the main menu isn't visible any more.
- JavaScript
- TypeScript
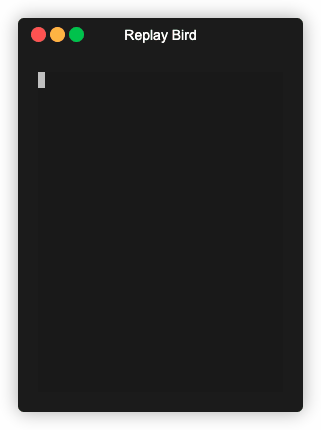