21 - Gameplay Test
Let's expand our test to play the game. We're going to check the bird can fly past 2 pipes, then crash into one, ending up with a score of 2
.
We pass an initRandom
field into testSprite
. This ensures device.random()
calls always produce the same result, in this case 0.5
, then 0.5
, then 0
. With this we can ensure our bird knows what height to fly at.
We create a function keepBirdInMiddle
which is going to keep clicking the screen when the bird's y
position is too low, to keep the bird jumping at the right height.
We can access the bird Texture through the getTexture("bird")
call in order to read its y
value. Here "bird"
is the testId
prop
which we need to pass into the t.image
Texture in our Bird
Sprite. The testId
prop can be passed into any Texture to reference it in a test.
We loop through the game's frames with jumpToFrame
, which will keep running until a condition returns either a Texture or true
. When that exits (bird hit a pipe!) we confirm the score shows as expected.
Lastly we can even confirm the sound effect played as expected after we crashed.
- JavaScript
- TypeScript
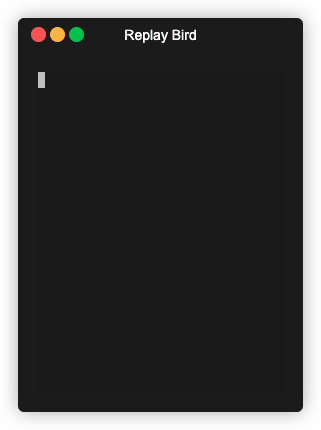